Quiz - 2020
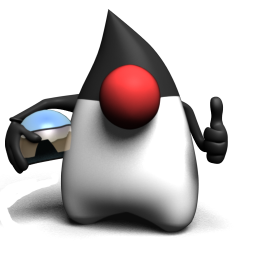
bogotobogo.com site search:
Reversing a string
package com.bogotobogo; public class Reverser { public String reverse(String input) { /* Method A * return new StringBuilder(input).reverse().toString(); */ /* Method B */ char[] a = input.toCharArray(); String rev = ""; for(int i = a.length-1; i >= 0;i--) rev += a[i]; return rev; } public static void main (String[] args) { System.out.println("in="+args[0]); System.out.println("out="+new Reverser().reverse(args[0])); } }
Output:
in=123456 out=654321
Samples of Array & ArrayList
Here is an array example: how to create and to pass into a method:
import java.util.*; public class test { public static void main(String[] args) { System.out.println("Binary search"); (new test()).go(); } public void go() { int[] array = new int[]{0,1,2,3,4,5,6,7,8,9}; int x = BinarySearch(array, 4); if(x == -1) System.out.println(x+" is not in the array!"); else System.out.println(x+" is at " + x); } public int BinarySearch(int[] a, int n) { int upper = a.length - 1; int lower = 0; int mid = 0; while(lower <= upper) { mid = (upper + lower)/2; if(n < a[mid]) upper = mid - 1; else if(n > a[mid]) lower = mid + 1; else return mid; } return -1; } }
Output:
Binary search 4 is at 4
The following code is a slightly modified version using ArrayList
// test.java package com.bogo; import java.util.ArrayList; public class test { public static void main(String[] args) { System.out.println("Binary search"); (new test()).go(); } public void go() { ArrayList<Integer> array = new ArrayList<Integer>(); for(int i = 0; i < 10; i++) { array.add(i); } Algorithms al = new Algorithms(); int x = al.BinarySearchArrayList(array, 4); if(x == -1) System.out.println(x+" is not in the array!"); else System.out.println(x+" is at " + x); } } // Algorithms.java package com.bogo; import java.util.ArrayList; public class Algorithms { public int BinarySearch(int[] a, int n) { int upper = a.length - 1; int lower = 0; int mid = 0; while(lower <= upper) { mid = (upper + lower)/2; if(n < a[mid]) upper = mid - 1; else if(n > a[mid]) lower = mid + 1; else return mid; } return -1; } public int BinarySearchArrayList(ArrayList<Integer> a, int n) { int upper = a.size() - 1; int lower = 0; int mid = 0; while(lower <= upper) { mid = (upper + lower)/2; if(n < a.get(mid)) upper = mid - 1; else if(n > a.get(mid)) lower = mid + 1; else return mid; } return -1; } }
String Permutation
//test.java package com.bogotobogo; public class test { public static void main(String[] args) { System.out.println("String Permutation"); (new test()).go(); } public void go() { String s = "1234"; Permutations(s); } public void Permutations(String s) { int length = s.length(); boolean[] used = new boolean[length]; char[] input = s.toCharArray(); StringBuffer output = new StringBuffer(); int level = 0; DoPermutations(input, output, used, length, level); } public void DoPermutations(char[] input, StringBuffer output, boolean[] used, int length, int level) { if(length == level) { System.out.println(output); return; } for(int i = 0; i < length; i++) { if(used[i] == true) continue; output.append(input[i]); used[i] = true; DoPermutations(input, output, used, length, level+1); used[i] = false; output.setLength(output.length()-1); } } }
Output is:
String Permutation 1234 1243 1324 1342 1423 1432 2134 2143 2314 2341 2413 2431 3124 3142 3214 3241 3412 3421 4123 4132 4213 4231 4312 4321
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization