From HTML to AngularJS
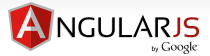
The HTML file below is a static HTML file. It acts as a placeholder for the basic functionality that we want to create.
<!DOCTYPE html> <html data-ng-app> <head> <title>One Two Three</title> <link href="bootstrap/css/bootstrap.css" rel="stylesheet" /> <link href="bootstrap/css/bootstrap-theme.css" rel="stylesheet" /> </head> <body> <div class="page-header"> <h1>Bogo's Table</h1> </div> <div class="panel"> <div class="input-group"> <input class="form-control" /> <span class="input-group-btn"> <button class="btn btn-default">Add</button> </span> </div> <table class="table table-striped"> <thead> <tr> <th>English</th> <th>Spanish</th> </tr> </thead> <tbody> <tr><td>One</td><td>Uno</td></tr> <tr><td>Two</td><td>Dos</td></tr> <tr><td>Three</td><td>Tres</td></tr> <tr><td>Four</td><td>Cuatro</td></tr> </tbody> </table> </div> </body> </html>
Here is the rendered page:
In the sections that follow, we're going to add AngularJS and use some basic features to bring us the application to life.
It is not difficult to add AngularJS to an HTML file. We simply add a script element to import the angular.js file, create an AngularJS module, and apply an attribute to the html element.
The arguments to the angular.module method are the name of the module to create and an array of other modules that are going to be needed. We have created a module called oneTwoThreeApp.
The AngularJS library dynamically compiles the HTML in a document in order to locate and process these additions and create an application. We can supplement the built-in functionality with JavaScript code to customize the behavior of the application and define our own additions to HTML.
<!DOCTYPE html> <html data-ng-app="onetwothreeApp"> <head> <title>One Two Three</title> <link href="bootstrap/css/bootstrap.css" rel="stylesheet" /> <link href="bootstrap/css/bootstrap-theme.css" rel="stylesheet" /> <script src="angular.js"></script> <script> var onetwothreeApp = angular.module("onetwothreeApp", []); </script> </head> <body> <div class="page-header"> <h1>Bogo's Table</h1> </div> <div class="panel"> <div class="input-group"> <input class="form-control" /> <span class="input-group-btn"> <button class="btn btn-default">Add</button> </span> </div> <table class="table table-striped"> <thead> <tr> <th>English</th> <th>Spanish</th> </tr> </thead> <tbody> <tr><td>One</td><td>Uno</td></tr> <tr><td>Two</td><td>Dos</td></tr> <tr><td>Three</td><td>Tres</td></tr> <tr><td>Four</td><td>Cuatro</td></tr> </tbody> </table> </div> </body> </html>
AngularJS compilation isn't like the compilation you might have encountered in C# or Java projects, where the compiler has to process the source code in order to generate output that the runtime can execute. It would be more accurate to say that the AngularJS library evaluates the HTML elements when the browser has loaded the content and uses standard DOM API and JavaScript features to add and remove elements, sets up event handlers, and so on.
There is no explicit compilation step in AngularJS development. AngularJS just modify our HTML and JavaScript files and load them into the browser.
Here is the rendered page with no difference from the previous one:
AngularJS supports the Model-View-Controller (MVC) pattern which requires us to break up the application into three distinct areas: the data in the application (the model), the logic that operates on that data (the controllers), and the logic that displays the data (the views).
Our first task is to pull all of the data together and separate it from the HTML elements in order to create a model. Separating the data from the way that it is presented to the user is one of the key ideas in the MVC pattern.
Since AngularJS applications exist in the browser, we need to define our data model using JavaScript within a script element:
Here is the rendered page :
Controllers are created by calling the controller method on the Module object returned by calling angular.module, as demonstrated in the previous section. The arguments to the controller method are the name for the new controller and a function that will be invoked to define the controller functionality.
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization