The core - Image Load, Convert, and Save
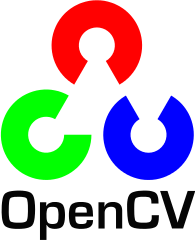
In this chapter, we'll learn how to load an image using imread().
Handling images is the basic building blocks of the OpenCV library and we should understand how to manipulate the images on a pixel level.
Also, we'll transform the loaded image to Greyscale format using cvtColor(). Then, we'll save the transformed image in a file using imwrite().
Actually, this is borrowed from opencv.org. However, depending on the platform or OpenCV version, it seems that we need to make some tweaks to make it work.
I am using OpenCV 3.0.0-dev from git and I am on Ubuntu 13.01 64 bit.
To me, since this tutorial is a Hello World for OpenCV, I cannot skip.
Below are the two pictures generated by the tutorial code:
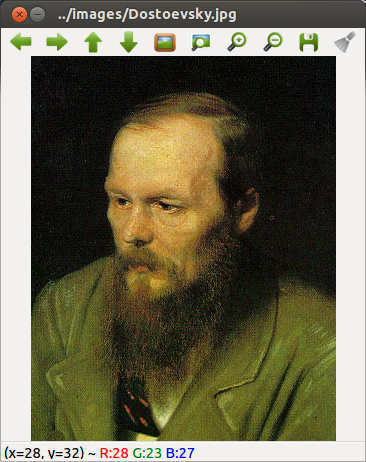
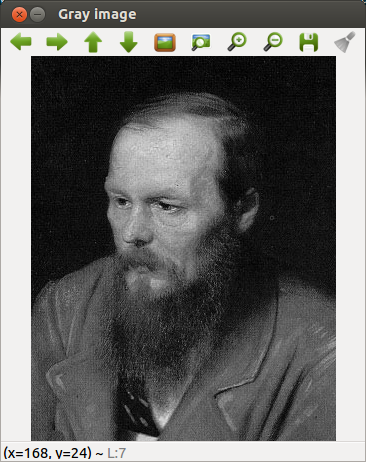
Here is our code, imgLoad.cpp:
#include <opencv2/opencv.hpp> #include <opencv2/highgui/highgui_c.h> using namespace cv; int main( int argc, char** argv ) { // argv[1] - whaere the image is located char* imagePath = argv[1]; // create a MAT object for input image Mat image; // load an image image = imread( imagePath, 1 ); if( argc != 2 || !image.data ) { printf( " No image data \n " ); return -1; } // create a MAT object for gray image Mat gray_image; // convert to Greyscale format // cvtColor( image, gray_image, CV_BGR2GRAY ); cvtColor( image, gray_image, COLOR_BGR2GRAY ); // save the transformed image to a file imwrite( "../images/GrayImage.jpg", gray_image ); // creates two windows namedWindow( imagePath, CV_WINDOW_AUTOSIZE ); namedWindow( "Gray image", CV_WINDOW_AUTOSIZE ); // imshow() displays an image in the specified window. // If the window was created with the CV_WINDOW_AUTOSIZE flag, // the image is shown with its original size imshow( imagePath, image ); imshow( "Gray image", gray_image ); // wait for key press waitKey(0); return 0; }
and with the cmake file, :
cmake_minimum_required(VERSION 2.8) project( ImageLoads ) find_package( OpenCV REQUIRED ) add_executable( ImageLoads imgLoad.cpp ) target_link_libraries( ImageLoads ${OpenCV_LIBS} )
So, put the two files in a directory called ImageLoads and run cmake:
$ cmake . -- Configuring done -- Generating done -- Build files have been written to: /home/khong/OpenCV/workspace/Tutorials/ImageLoads $ make Scanning dependencies of target ImageLoads [100%] Building CXX object CMakeFiles/ImageLoads.dir/imgLoad.cpp.o Linking CXX executable ImageLoads [100%] Built target ImageLoads
If we run the code, it will create two windows each of them has a picture as shown above.
$ ./ImageLoads ../images/Dostoevsky.jpg
The input image is available Dostoevsky.jpg.
- Initially, I got an error: "CV_WINDOW_AUTOSIZE was not declared in this scope."
It turned out I need "#include <opencv2/highgui/highgui_c.h> - I also got a similar error for 'CV_BGR2GRAY'
That was because of I'm using OpenCV 3.0.0 from git.
So, I modified it to 'COLOR_BGR2GRAY' which is defined in 'opencv2/imgproc.hpp' - The cvtColor() takes as arguments:
- a source image (image)
- a destination image (gray_image), in which we will save the converted image.
- an additional parameter that indicates what kind of transformation will be performed. In this case we use COLOR_BGR2GRAY (because of imread has BGR default channel order in case of color images).
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization